Hello, welcome to The Keypress.
In this post I'll be talking about my most recent App (still in development). This app was made at the Apple Developer Academy in a timespan of one week. The goal for this app was to learn something new. Really, anything at all.
Since this app was made in 7 days, I'm going to divide each day into it's own chapter
Monday – the beginning
It's Monday morning. I've finished my previous App presentation and wrapped up the final details related to it. We had 2 one-week apps back-to-back, it's really important to acknowledge when one cycle ends and when another begins.
We got our theme: Quiz App. I normally tend to have ideas very quickly but not this time. This time I had a creative block, so I mostly played with some basic ideas trying to find something that would engage me in.
I didn't really made anything else besides finding some basic references and playing Loldle
Tuesday – the idea
After playing a bit more of Loldle, I settled on making something similar. Loldle has 5 different puzzles about guessing champions. Taking a look at League of Legends, what felt as the next “logical” step was to find out what sort of things could be guessed based on their characteristics.
If you played even a tiny bit of League of Legends, you know the game features an absurdly big collection of items, designed to change your character stats and turn the tide of the match.
This was the “Aha” moment: a game in which you have to guess what is the LoL item, based on what stats they modify.
Enter the Rift API
Riot Games offer many different endpoints, but what interested me the most was Data Dragon. DD (for short) has an impressive collection of all items available in game and their stats (well, most would be more suited, more on that later). This API is offered without requiring any token and it's served as a JSON.
This JSON would contain all the data I need if not for some major inconsistencies. On a simple level, some fields have different names than their in-game counterparts: Magic Resistance is Spell Block; The file contains Arena items. More concerning problems are the Lack of some attributes: Magic Penetration is referenced in the stats block but doesn't show up in any item, the same with Ability Haste; Not all Ornn items are correctly flagged. These problems were very worrying, they seemed like the sort of thing I'd have to deal manually with each attribute.
At this point in time I started to feel behind schedule. How would I manually patch a list with more than 200 items? Time for a new helper: ChatGPT
ChatGPT in this case can help with converting my natural language description of the issue into usable python code that solves my problems.
I have a python dict with an object following this pattern: [...json sample] Using python, I need to map this into a list of dict. Each object in the dict should have the following properties: name, id (the key of the object), I need the into (if the dict doesnt contain into, create one empty), I need the playtext description (if it doesnt have a plaintext description I need the regular description without the <> tags ). I need the image property, but only the sprite and x,y,w,h. I also need the stats properties
stats is also a dict, I need to map some keys to specific names.
FlatPhysicalDamageMod to Attack Damage
FlatMagicDamageMod to Ability Damange
FlatHPPoolMod to Life
With the python code generated by ChatGPT and my manual fixes and adaptations, I was able to transform the raw API data into something that was useful for the App
Wednesday – despair
Going into Wednesday I had this lurking feeling of despair. I was halfway through the week but far away from an App. I turned to Figma in order to understand how I would structure the screens and data.
This is one point I thought I could do better than Loldle: their interfaces were designed for widescreens and weren't really optimal for mobile screens. I always thought that information didn't move consistently on their UI: The input field for guessing is above the guessed champions list then when the user tries to guess another champion, their screen moves up (to accomodate the keyboard) then there's the champion list between the keyboard and the text input and finally once you guess a champion, the guesses list grows down.
In my opinion this made the game behave weirdly on mobile devices (and because of that I tend to play only on PC).
The way I tackled these issues was to start sorting where things would appear on screen (from the bottom up): Keyboard, text input, list of items (based on what is written in the text input), then list of items that were already guessed and finally the Hints. With that out of the way I added some colors based on the game itself: Blue and Red for the background, a brown-ish tone for the boxes and a golden yellow for the frames and text.
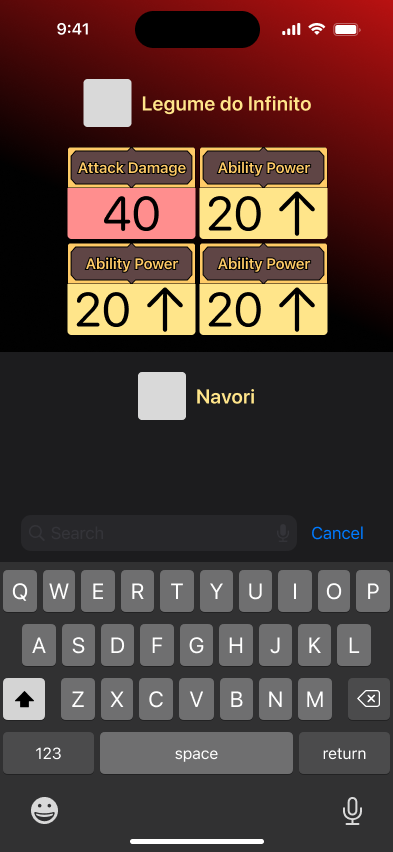
I made the frames on the objects as a Shape in swift. In other words It's a vector that automatically scales into the container size, which makes it very responsive. The last thing was to display an Item on the screen. This took some effort as the item image is contain in an Atlas with all the other similar items. By the end of the day I had a basic UI with a list of items being displayed
Thursday – when it all comes together
My second to last day saw the most progress.
I added the guessing mechanic, I added the item grid display and implemented the hints system. At this point in time I started play testing with friends and for my amusement the game was fun, what a relief.
But then we stumbled upon something weird, some items were duplicated and there were some items with different names. At this point in time a good knowledge of the game was very handy because this was no mistake in the API, rather these were Arena and Ornn items. Once again I turned to python to fix these issues (and later I found out I still didn't fix all of those, thanks Riot)
I also decided at this point in time that Localization would be great. And to my surprise Xcode and Swift are pretty great when it comes to localization, in less than 10 minutes I had my entire app localized to both Portuguese and English. To Localize the Items I had 2 JSON files, one in each language. This was also easy thanks to Riot providing their Item API in multiple languages.
With the app almost done it was time for the final steps
Friday – thank god it's friday
The final day started well, with most issued fixed all I had to do was add the game logic to restart once the player figures out which item it was. With that out of the way I turned to IAPs.
While I dislike intrusive Ads, I think IAPs when used correctly are a great way to generate income with the App and allow the user to do new things. My strategy was quite simple: by default the game has items related to Attack Damage, Ability Power and Life. The IAPs available allow the user to access new items sets such as Armor and Magic Resistance. This also came with a feature to enable and disable item sets. The user can play the game with any set configuration they think is better.
And for the final day I also released a test version over on Test Flight, which you can access here
Wrap up
In conclusion I'm very happy with what I achieved. I think this game has potential within the League of Legends community not only as a fun game but also as a way to develop a better understanding of the game items and builds. While there's no release date yet, I'm hopping for sometime until December.
Thank you for reading!